IOS Programming Part 3 (Moving object around the screen when user touch
- Tua
- Apr 15, 2016
- 2 min read
For this lesson, we are going to learn how to make object moving around the screen when user touch on any where on the screen. Let have fun together.
Step1: Create a project in Xcode and select a single view type
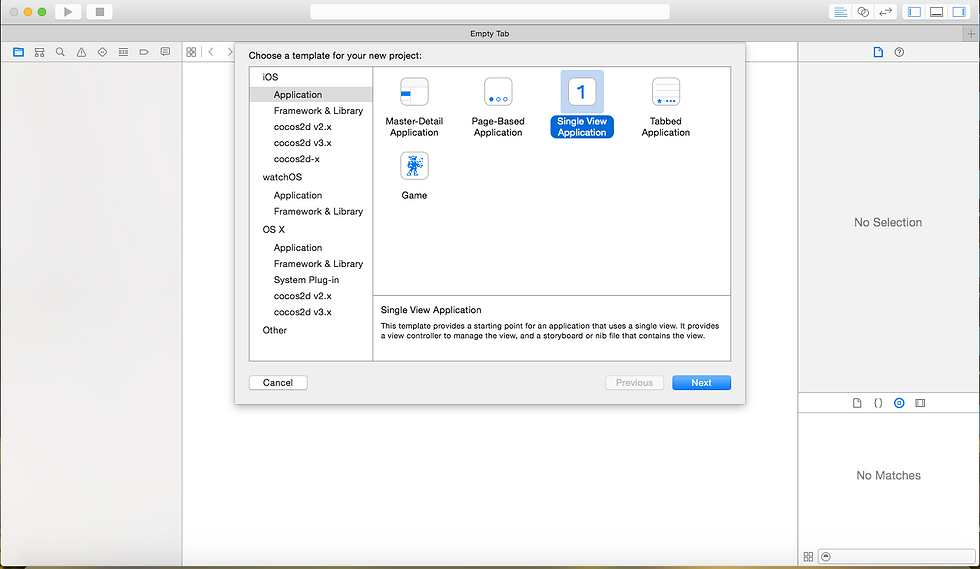
Step2: Then adding imgview object to your screen by dragging it from object library and placing it on the screen as shown below
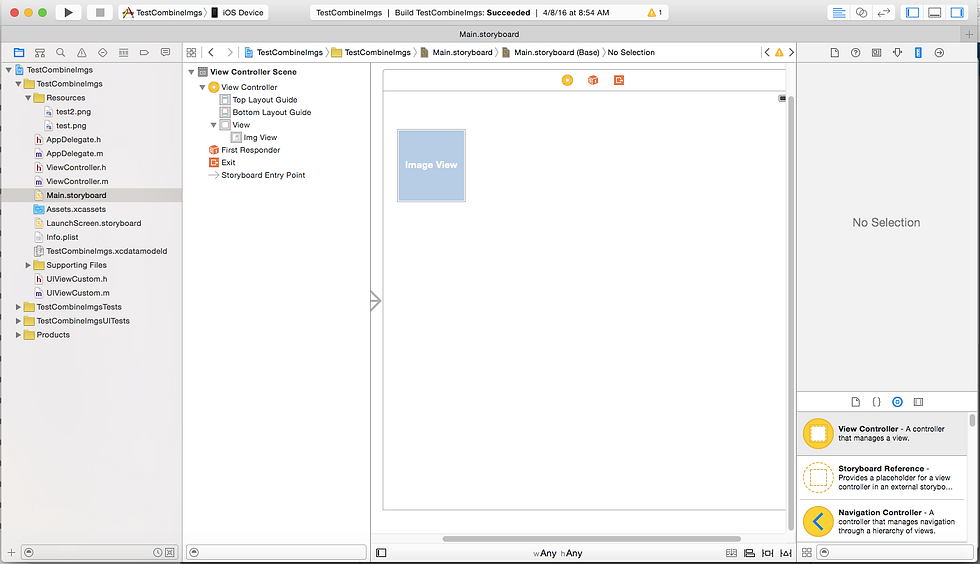
Step3: open viewcontroller.h and add the following code
@interface ViewController : UIViewController{
UIView *m_CurrentView;
BOOL m_MoveDone;
CGPoint m_Dest;
CGPoint m_Pos;
NSTimer *m_TimerPtr;
}
@property (strong, nonatomic) IBOutlet UIImageView *imgView;
-(void) MoveToDestination;
Step4: Now, It is time to add some code to make it work. Let start with open your viewcontroller.cpp, then add the following code in viewdidload method.
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
NSString *thePath = [[NSBundle mainBundle] pathForResource:@"test" ofType:@"png"];
UIImage *img = [[UIImage alloc] initWithContentsOfFile:thePath];
m_CurrentView = nil;
_imgView.image = img;
m_TimerPtr = nil;
}
Now, we will see it step by step how the code above work.
First, we create NSString object to receive to path to our image file. Then we create UIImage object (img2) to receive the image read from the path. Lastly, we set the m_TimerPtr which is NSTimer object to nil.
Step5: Now, it come to the important part, inside TouchBegan method, add the following code
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
UITouch *touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
m_Dest = location;
if(m_TimerPtr != nil){
[m_TimerPtr invalidate];
m_TimerPtr = nil;
}
m_TimerPtr = [NSTimer scheduledTimerWithTimeInterval:0.05f
target:self
selector:@selector(MoveToDestination)
userInfo:nil
repeats:TRUE];
}
The code begin with keeping touch location to m_Dest variable and then it check to see if m_TimerPtr has been created already. If so the code release it and recreate it again whenever user touch on the screen. Then the timer is used to control method MoveToDestination which is used to make the object move to touched point. More information about this method will be explained later in the next step.
Step6: Let take a look MoveToDestination method, the key method that is used to make object moving to the touched point
-(void) MoveToDestination{
CGPoint p = _imgView.frame.origin;
p.x = p.x + _imgView.frame.size.width * 0.5f;
p.y = p.y + _imgView.frame.size.height * 0.5f;
float dist = sqrtf(powf((p.x - m_Dest.x),2) + powf((p.y - m_Dest.y),2));
if(dist > 5.0f){
CGPoint vec;
vec.x = m_Dest.x - p.x;
vec.y = m_Dest.y - p.y;
if(dist > 0.0f){
vec.x /= dist;
vec.y /= dist;
vec.x *= 10.0f;
vec.y *= 10.0f;
}
m_Pos.x += vec.x;
m_Pos.y += vec.y;
CGAffineTransform t;
t = CGAffineTransformMakeTranslation(m_Pos.x,m_Pos.y);
[_imgView setTransform:t];
}
}
The code work by checking the distance between the touched point and the object position. If the distance is more than 5.0 units then it continue to calculate the vector direction between them by subtracting touched point with object position, normalize the calculated vector and then apply it to the current position of the object. Lastly, create CGAffineTransfrom variable and set it's value to updated object position and then call method setTranform of the object to move. Build and Run your project. Then you should see the object moving around whenever you touch on the screen.
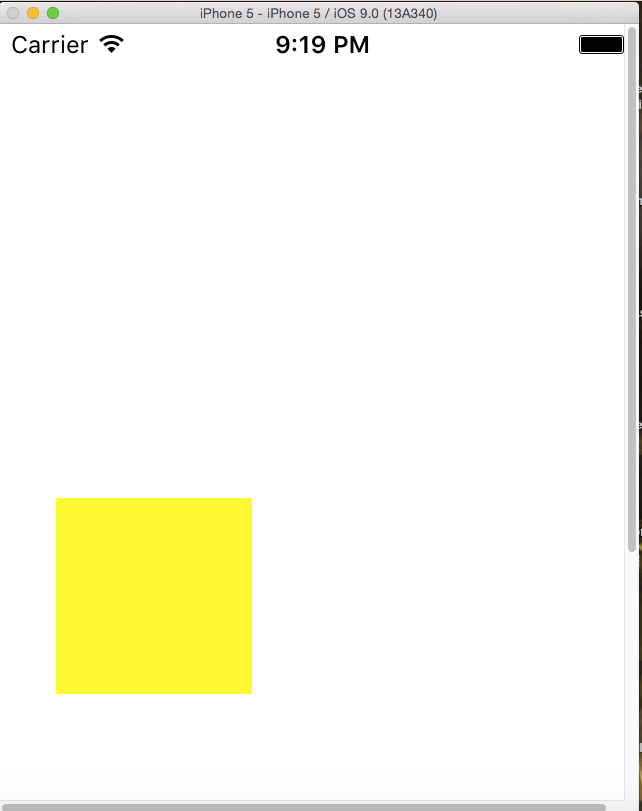
Comments